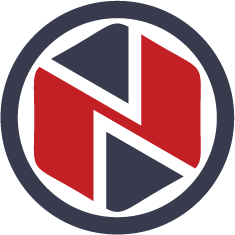
When: 2022
Why: To make your Ionic/Angular App support multiple languages
Time: 30 minutes
Tags: Ionic, Angular, ngx
The ngx-translate/core library
NGX-Translate is an internationalization library for Angular. It lets you define translations for your content in different languages and switch between them easily. Check out the demo on StackBlitz.
It gives you access to a service, a directive and a pipe to handle any dynamic or static content.
NGX-Translate is also extremely modular. It is written in a way that makes it really easy to replace any part with a custom implementation in case the existing one doesn’t fit your needs.
1. Check what angular version you have:
ng version
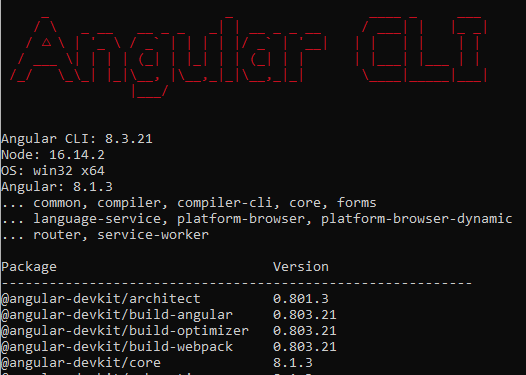
2. Choose the version corresponding to your Angular version:
Angular | @ngx-translate/core | @ngx-translate/http-loader |
---|---|---|
13 (ivy only) | 14.x+ | 7.x+ |
10/11/12/13 | 13.x+ | 6.x+ |
9 | 12.x+ | 5.x+ |
8 | 12.x+ | 4.x+ |
7 | 11.x+ | 4.x+ |
6 | 10.x | 3.x |
5 | 8.x to 9.x | 1.x to 2.x |
4.3 | 7.x or less | 1.x to 2.x |
2 to 4.2.x | 7.x or less | 0.x |
You can check the availables versions here:
https://github.com/ngx-translate/core/releases
In my case, i will install the latest version of @ngx-translate/core for Angular 8.0:
npm install @ngx-translate/core@12.1.2 --save
You can review you package.json file after run the last command:
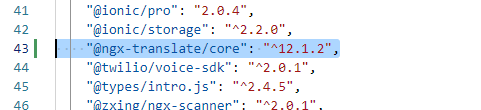
2. Add the library @ngx-translate/http-loader to “load” your translations files, you can view all the availables versions here:
https://github.com/ngx-translate/http-loader/releases
npm install @ngx-translate/http-loader@4.0.0 --save
You can review you package.json file after run the last command:
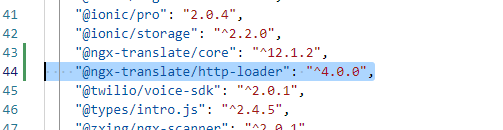
3. Edit app.module:
In this example, we will create the translation files in the folder assets/i18n.
//required libraries for translation
import { HttpClient} from '@angular/common/http';
import { TranslateModule, TranslateLoader } from '@ngx-translate/core';
import { TranslateHttpLoader } from '@ngx-translate/http-loader';
// required for AOT compilation
export function LanguageLoader(http: HttpClient) {
return new TranslateHttpLoader(http, 'assets/i18n/', '.json');
}
@NgModule({
imports: [
TranslateModule.forRoot({
loader: {
provide: TranslateLoader,
useFactory: (LanguageLoader),
deps: [HttpClient]
}
}), ...
4. Edit the component module where you want the translation pipe.
import {TranslateModule} from '@ngx-translate/core';
@NgModule({
imports: [
TranslateModule,
...
],
This could be residente-main.module.ts
5. Add the translation service at the component page where you are going to make the translation:
import { TranslateService } from "@ngx-translate/core";
@Component({
selector: 'residente-main',
templateUrl: 'residente-main.page.html',
styleUrls: ['residente-main.page.scss']
})
export class ResidenteMainPage {
constructor(
public translate: TranslateService) {
translate.setDefaultLang('es');
translate.use('es');
...
This could be residente-main.page.ts. Whe defined default language as english (“en”), but we switch to espanish (“es”).
6. Create the translation files in the directory assets/i18n
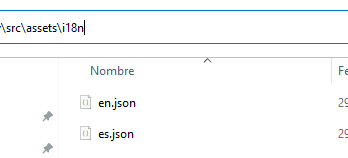
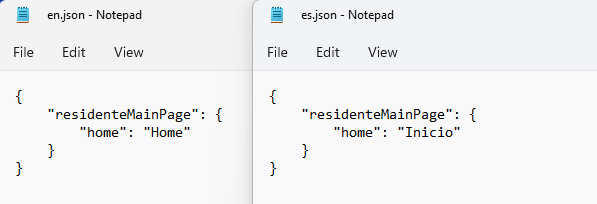
7. Now you can use the translation pipe in your component html file:
<ion-label> {{ 'residenteMainPage.home' | translate }} </ion-label>
This could by residente-main.page.html
8. Use the translation in the ts file.
If you need to use the translation in the ts file, you can get the translation using then method get() in the constructor.
import { TranslateService } from "@ngx-translate/core";
@Component({
selector: 'residente-main',
templateUrl: 'residente-main.page.html',
styleUrls: ['residente-main.page.scss']
})
export class ResidenteMainPage {
public translation: any;
constructor(
public translate: TranslateService) {
translate.setDefaultLang('es');
translate.use('es');
this.translateSubscription = translate.onLangChange.subscribe(() => {
translate.get('residenteMainPage').toPromise().then((resp: any) => {
this.translation= resp;
});
});
...
As you can see, we firts subscribe to detect any language changes.
Now you can use the translation in your code:
console.log(this.translation.home);